In this blog, we will be going to learn about how to create a desktop application using angular and electron,
and Yes angular and electron are both different things whereas angular is a frontend MVC framework that is used to build things related to frontend only and ELectron is an npm package from which we can create a desktop application using angular and for now using Electron creating desktop application becomes easy and smooth and no complexity is there to create a desktop application we just need to follow some sort of steps and that’s it.
So one of the questions everyone had in their mind is what will be the prerequisite for creating desktop apps using angular, So below mentioned things are needed or some sort of knowledge is required related to that,
1. HTML, CSS, and Javascript.
2. Node and Npm.
3. Some TypeScript Knowledge is sufficient.
4. Angular.
5. Visual Studio Code for Development Purpose
Let’s discuss why these things are needed to create a desktop application. So HTML, CSS, and Javascript is used to create components, User Interface, and dynamic pages. Only basic knowledge is required for that. For Node and Npm we must know how to install packages using the npm install command and how node modules works will be good info. For angular, we must know what are the components and how MVC works.
Let’s Get Started with the creation of desktop applications using angular.
For Creating Desktop Applications we have to follow Below Steps.
Step1: Initialize Angular in your favorite folder by entering the below command.
– npm install -g @angular/cli@latest
this command will initialize the development environment for angular.

Step2: Create a new angular app By entering the Below Command.
-ng new create-desktop-app –no-strict.
the above command will create a new desktop app with the name create-desktop-app.

after running the above command some files will be initialized under the create-desktop-app folder as shown below.
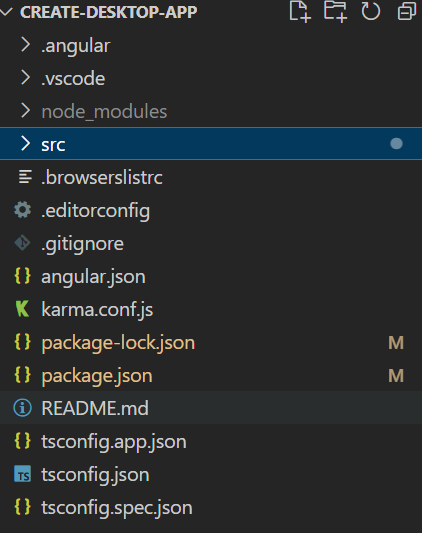
Step3: After create-desktop-app we have to install the electron package inside that folder only, So type the below command to enter the run cmd command in that folder.
-cd create-desktop-app

-After entering into the development folder install the electron package by entering the below command.
-npm install electron –save-dev
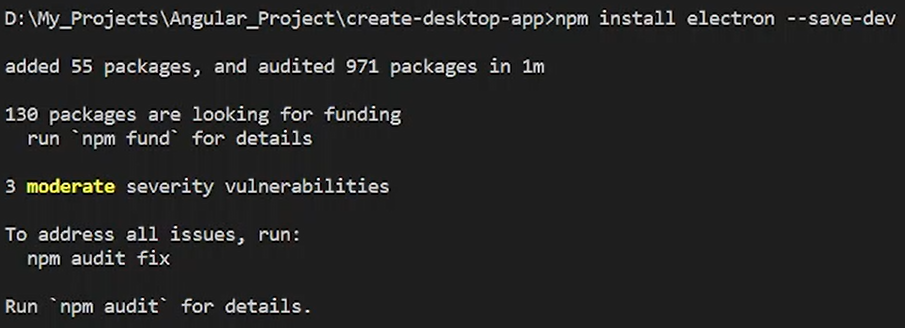
The above command will install the required package of electrons from which we can do the further process.
Step4: Create electron.dev.js file under create-desktop-app/src folder and paste the below code
-filename: electron.dev.js
const { app, BrowserWindow } = require('electron');
const path = require('path');
const url = require('url');
// Keep a global reference of the window object, if you don't, the window will
// be closed automatically when the JavaScript object is garbage collected.
let win;
const createWindow = () => {
// set timeout to render the window not until the Angular
// compiler is ready to show the project
setTimeout(() => {
// Create the browser window.
win = new BrowserWindow({
width: 800,
height: 600,
icon: './src/favicon.ico'
});
// and load the app.
win.loadURL(url.format({
pathname: 'localhost:4200',
protocol: 'http:',
slashes: true
}));
win.webContents.openDevTools();
// Emitted when the window is closed.
win.on('closed', () => {
// Dereference the window object, usually you would store windows
// in an array if your app supports multi windows, this is the time
// when you should delete the corresponding element.
win = null;
});
}, 10000);
}
// This method will be called when Electron has finished
// initialization and is ready to create browser windows.
// Some APIs can only be used after this event occurs.
app.on('ready', createWindow);
// Quit when all windows are closed.
app.on('window-all-closed', () => {
// On macOS it is common for applications and their menu bar
// to stay active until the user quits explicitly with Cmd + Q
if (process.platform !== 'darwin') {
app.quit();
}
});
app.on('activate', () => {
// On macOS it's common to re-create a window in the app when the
// dock icon is clicked and there are no other windows open.
if (win === null) {
createWindow();
}
});
The above code is used to create a desktop window after running the angular application from which the desktop app is created at runtime.
Step5: We have to do some changes in the package.json folder but before doing changes we have to install a concurrently package to run angular and electron at the same time. So to install that write the below command.
-npm install concurrently –save-dev
this will install the concurrently package and after that, we have to do some changes inside the package.json folder inside create-desktop-app.
The main changes we have to do inside the scripts section inside package.json:
because of adding the electron package, we have to add an electron key inside the scripts
like "electron": "electron ./src/electron.dev"
and another thing we have to do is to add things under start like "start": "concurrently \"ng serve\" \"npm run electron\""
,
for your reference, you can compare the packages.json folder with your folder
file: package.json:
{
"name": "create-desktop-app",
"version": "0.0.0",
"scripts": {
"ng": "ng",
"start": "concurrently \"ng serve\" \"npm run electron\"",
"electron": "electron ./src/electron.dev",
"build": "ng build",
"watch": "ng build --watch --configuration development",
"test": "ng test"
},
"private": true,
"dependencies": {
"@angular/animations": "^14.2.0",
"@angular/common": "^14.2.0",
"@angular/compiler": "^14.2.0",
"@angular/core": "^14.2.0",
"@angular/forms": "^14.2.0",
"@angular/platform-browser": "^14.2.0",
"@angular/platform-browser-dynamic": "^14.2.0",
"@angular/router": "^14.2.0",
"rxjs": "~7.5.0",
"tslib": "^2.3.0",
"zone.js": "~0.11.4"
},
"devDependencies": {
"@angular-devkit/build-angular": "^14.2.6",
"@angular/cli": "~14.2.6",
"@angular/compiler-cli": "^14.2.0",
"@types/jasmine": "~4.0.0",
"concurrently": "^7.4.0",
"electron": "^21.1.1",
"jasmine-core": "~4.3.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.1.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.0.0",
"typescript": "~4.7.2"
}
}
Step 6: Now everything is done to run our desktop application and now we can write the below command to run.
-npm start
After running this command one window will open which is our desktop application as shown in the below image.
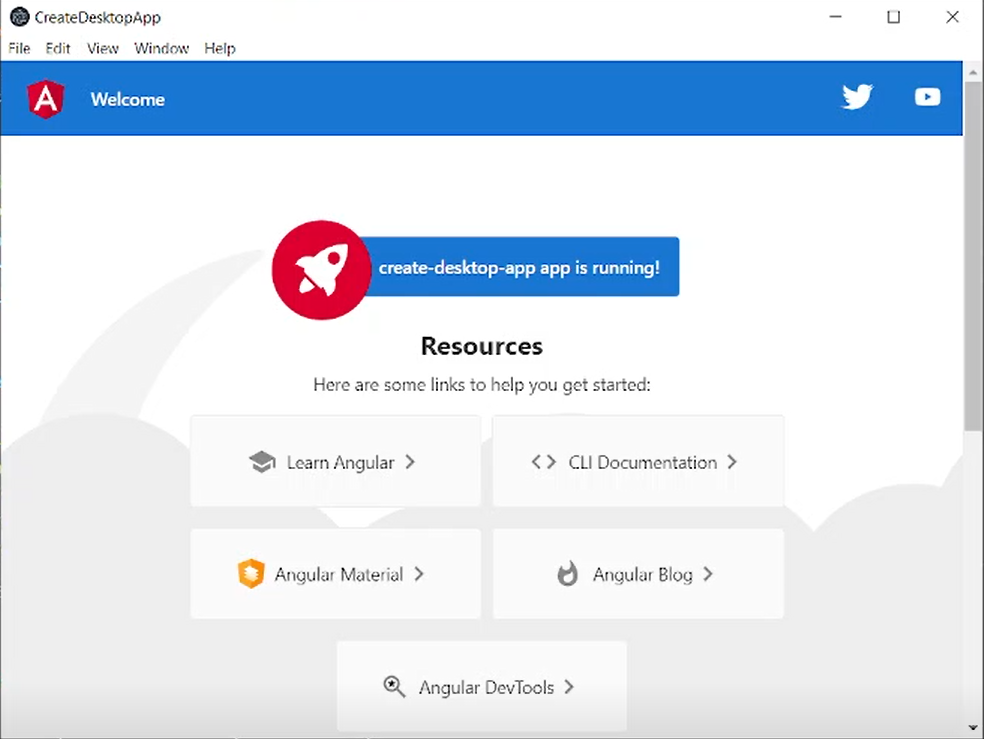
For more reference Watch Below Mentioned Video.